Configurable handlers with PHP attributes
Contributed by Alireza Mirsepassi
in #43588.
PHP attributes are a great way of adding metadata to PHP code. In Symfony we're adding the option of using PHP attributes to configure most things. That's why in Symfony 5.4 we're allowing to configure message handlers with attributes. Instead of having to implement the MessageHandlerInterface, you can now add the AsMessageHandler attribute to any PHP class and use it as a message handler:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 // src/MessageHandler/SmsNotificationHandler.php namespace App\MessageHandler;
use App\Message\OtherSmsNotification; use App\Message\SmsNotification; use Symfony\Component\Messenger\Attribute\AsMessageHandler;
[AsMessageHandler(fromTransport: 'async', priority: 10)]
class SmsNotificationHandler { public function __invoke(SmsNotification $message) { // ... } }
Worker metadata
Contributed by Oleg Krasavin
in #42335.
Currently there's no simple way to get worker metadata such as its transport name. In Symfony 5.4 we're improving this with the introduction of a new WorkerMetadata class which is accessible via $worker->getWorkerMetadata(). For example, inside a method of some listener/subscriber that handles Symfony Messenger events you could use something like this:
1
2 3 4 5 6 7 8 9 public function resetServices(WorkerRunningEvent $event): void { $actualTransportName = $event->getWorker()->getWorkerMetadata()->getTransportName(); if (!$event->isWorkerIdle() || !in_array($actualTransportName, $this->receiversName, true)) { return; }
$this->servicesResetter->reset();
}
Reset container services between messages
Contributed by Grégoire Pineau
in #41163
and #43322.
Container services are not reset automatically when handling messages. This can be a problem for example with the Monolog fingers crossed handler. Since services are not reset, if the first message triggers an error, the next messages will log and ultimately overflow the buffer. In Symfony 5.4 we're improving this situation with the option to automatically reset services after handling a message. To use this feature, set the new reset_on_message option to true in your messenger configuration:
1
2 3 4 5 6 7 8 9
config/packages/messenger.yaml
framework: messenger: transports: async: dsn: '%env(MESSENGER_TRANSPORT_DSN)%' reset_on_message: true failed: 'doctrine://default?queue_name=failed' sync: 'sync://'
Handle messages in batches
Contributed by Nicolas Grekas
in #43354.
Sometimes, when using the Messenger component, you could handle multiple messages at once instead of processing them one by one. In Symfony 5.4 we've introduced a new BatchHandlerInterface that allows your handlers to process messages in batches. Handlers implementing this interface should expect a new $ack optional argument to be provided when invoke() is called. If you don't provide the $ack argument, the message is handled synchronously as usual. If you provide $ack, invoke() is expected to buffer the message and its $ack function, and to return the number of pending messages in the batch. Here is what a batch handler might look like:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 class MyBatchHandler implements BatchHandlerInterface { use BatchHandlerTrait;
public function __invoke(MyMessage $message, Acknowledger $ack = null)
{
return $this->handle($message, $ack);
}
private function process(array $jobs): void
{
foreach ($jobs as [$job, $ack]) {
try {
// [...] compute $result from $job
$ack->ack($result);
} catch (\Throwable $e) {
$ack->nack($e);
}
}
}
}
The size of the batch is controlled by BatchHandlerTrait::shouldProcess() (defaults to 10).
Sponsor the Symfony project.
Login to add comment
Other posts in this group
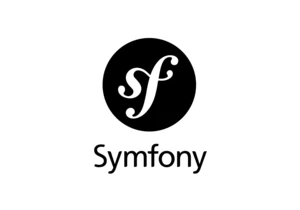
This week, Symfony unveiled the Symfony AI initiative, a set of components and bundles designed to bring powerful AI capabilities directly into your PHP applications. In addition, we published travel
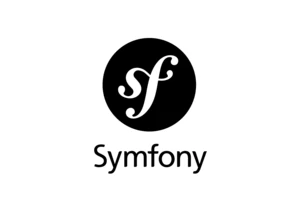
Today we are happy to announce a new Symfony initiative called Symfony AI - with the goal to provide a comprehensive set of components and bundles designed to bring powerful AI capabilities directly i
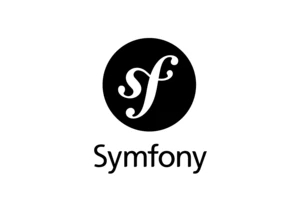
SymfonyCon Amsterdam 2025, our next annual international Symfony conference, will take place on:
November 25 & 26: 2 workshops days with several topics to learn, practice and improve your skills
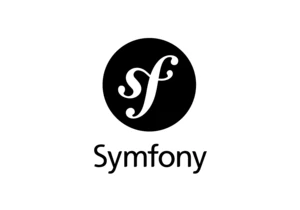
This week, development on the upcoming Symfony 8.0 version continued with the removal of deprecated features and the marking of several classes as final. In addition, we published two new case studies
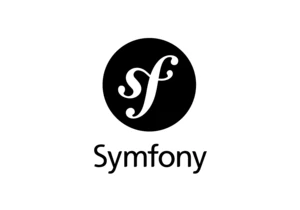
At Wide, Micropole’s digital agency, they help leading brands modernize their digital infrastructures while ensuring scalability, security, and performance. When Audi France approached them to migrate
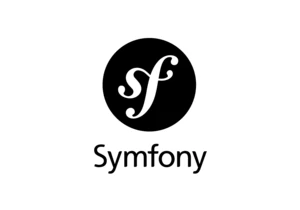
Vente-unique.com, a leading European online retailer of furniture and home decor, operates in 11 countries, powered by a team of 400 professionals and serving more than 3 million customers. From 15 ye
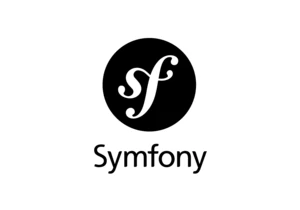
This week, Symfony 6.4.23, 7.2.8 and 7.3.1 maintenance versions were released. Meanwhile, the upcoming Symfony 7.4 version continued adding new features such as better controller helpers, more precisi