The Symfony Validator component includes some advanced constraints such as Callback (to implement custom validation rules), Sequentially (to apply a set of rules in order and interrupt them at any point), Compound (to create a set of reusable constraints), etc.
In Symfony 6.2 we're adding another advanced constraint to that list: When
,
which allows to implement conditional validations.
Consider a Discount
class with two properties:
// src/Model/Discount.php
namespace App\Model;
class Discount
{
private ?string $type;
private ?int $value;
// ...
}
To validate the object contents, you need to apply these rules:
- If
type
ispercent
, thenvalue
must be less than or equal100
; - If
type
isabsolute
, thenvalue
can be any value; - In all cases, the
value
must be greater than 0.
The new When
constraint defines tow main options called expression
and
constraints
. These constraints are only enforced when the result of evaluating
the expression is true
. You can use it as follows to validate that the
value
is less than 100
only if the discount type
is percent
:
use Symfony\Component\Validator\Constraints as Assert;
// ...
class Discount
{
#[Assert\GreaterThan(0)]
#[Assert\When(
expression: 'this.type == "percent"',
constraints: [
new Assert\LessThanOrEqual(100, message: 'The value should be between 1 and 100!')
],
)]
private ?int $value;
// ...
}
The condition passed to the expression
option must use the Symfony
ExpressionLanguage syntax. Inside the expression you can use the this
variable to refer to the object being validated and value
to refer to the
property being valuated (this is only available if you apply the When
constraint to properties).
Finally, you can combine When
with other advanced constraints such as Callback
to define complex conditional validations:
use Symfony\Component\Validator\Constraints as Assert;
use Symfony\Component\Validator\Context\ExecutionContextInterface;
class Discount
{
#[Assert\When(
expression: 'value == "percent"',
constraints: [new Assert\Callback('doComplexValidation')],
)]
private ?string $type;
// ...
}
<hr style="margin-bottom: 5px" />
<div style="font-size: 90%">
<a href="https://symfony.com/sponsor">Sponsor</a> the Symfony project.
</div>
Accedi per aggiungere un commento
Altri post in questo gruppo
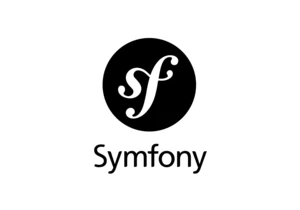
This week, Symfony unveiled the Symfony AI initiative, a set of components and bundles designed to bring powerful AI capabilities directly into your PHP applications. In addition, we published travel
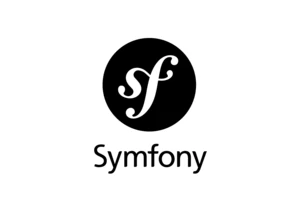
Today we are happy to announce a new Symfony initiative called Symfony AI - with the goal to provide a comprehensive set of components and bundles designed to bring powerful AI capabilities directly i
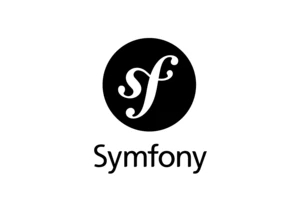
SymfonyCon Amsterdam 2025, our next annual international Symfony conference, will take place on:
November 25 & 26: 2 workshops days with several topics to learn, practice and improve your skills
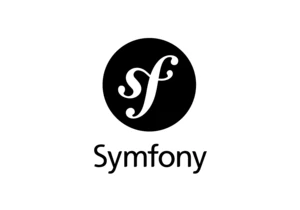
This week, development on the upcoming Symfony 8.0 version continued with the removal of deprecated features and the marking of several classes as final. In addition, we published two new case studies
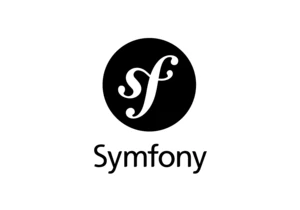
At Wide, Micropole’s digital agency, they help leading brands modernize their digital infrastructures while ensuring scalability, security, and performance. When Audi France approached them to migrate
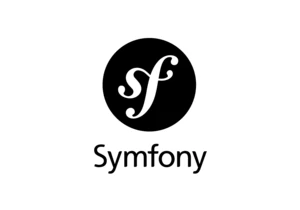
Vente-unique.com, a leading European online retailer of furniture and home decor, operates in 11 countries, powered by a team of 400 professionals and serving more than 3 million customers. From 15 ye
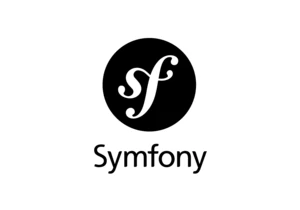
This week, Symfony 6.4.23, 7.2.8 and 7.3.1 maintenance versions were released. Meanwhile, the upcoming Symfony 7.4 version continued adding new features such as better controller helpers, more precisi