
A complete guide to the Riverpod package for Flutter state management. Included: core concepts & how to use all the available providers. https://codewithandrea.com/videos/flutter-state-management-riverpod/
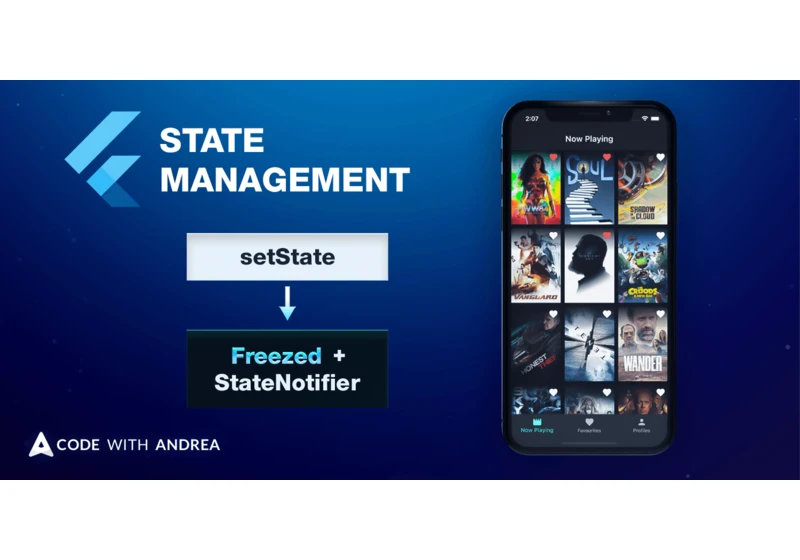
Mixing UI and logic inside Flutter widgets is bad. Here's how to refactor a simple app for better separation of concerns, immutability, and type safety using Freezed & State Notifier. https://codewithandrea.com/videos/flutter-state-management-setstate-freezed-state-notifier-provider/
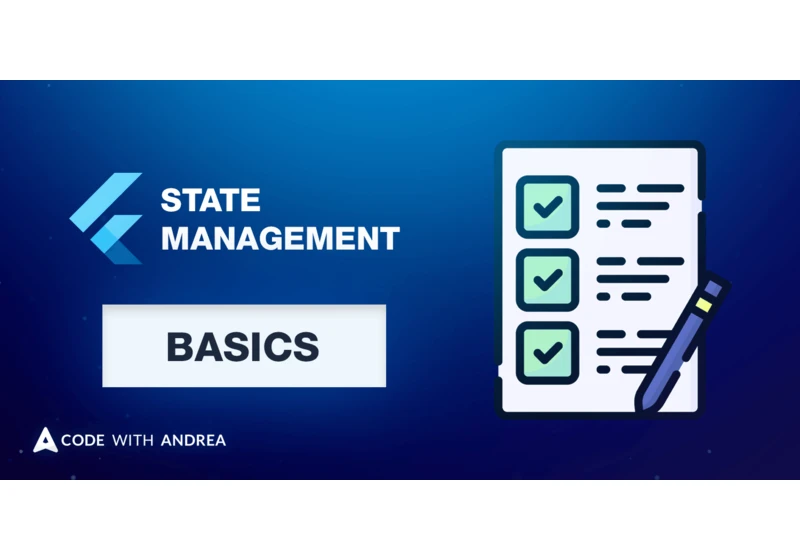
An overview of Flutter's built-in widgets for managing state, along with links to the best resources from the official Flutter documentation. https://codewithandrea.com/videos/flutter-state-management-basics/

Two effective techniques for reducing code generation times for Flutter apps that use with build_runner. https://codewithandrea.com/tips/speed-up-code-generation-build-runner-dart-flutter/

Login and registration flow with Firebase. Part 2 shows how to build an email and password form with validation, and how to add Firebase to the project (iOS setup included). https://codewithandrea.com/videos/flutter-firebase-auth-part2/

Login and registration flow with Firebase. Part 3 shows how to sign in with Firebase authentication, add the registration flow, write asynchronous code with async/await, and handle errors. https://codewithandrea.com/videos/flutter-firebase-auth-part3/

Let's see how to use platform channels by building an image picker on iOS, and using it from the Dart code. https://codewithandrea.com/articles/platform-channels-flutter/

Login and registration flow with Firebase. Part 4 shows how to redirect user to a welcome screen after logging in. Also how to refactor the authentication code into a separate reusable class. https://codewithandrea.com/videos/flutter-firebase-auth-part4/

Login and registration flow with Firebase. Part 5 shows how to add a root widget and control what UI to show depending on authentication state. https://codewithandrea.com/videos/flutter-firebase-auth-part5/

Login and registration flow with Firebase. Part 6 shows how to create a home page and complete the sign out flow. https://codewithandrea.com/videos/flutter-firebase-auth-part6/