
How to style an ElevatedButton in Flutter, including reusing the same style across all buttons with ThemeData. https://codewithandrea.com/tips/elevated-button-style-flutter/
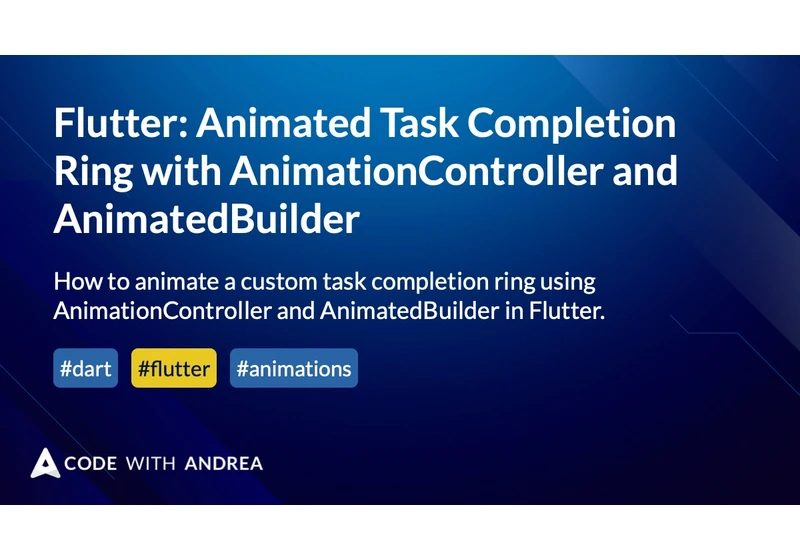
How to animate a custom task completion ring using AnimationController and AnimatedBuilder in Flutter. https://codewithandrea.com/articles/flutter-animation-controller-animated-builder/

CustomPainter is often the way to go when we need to draw some custom shapes in Flutter. Let's see how to use it in practice. https://codewithandrea.com/articles/flutter-drawing-with-custom-painter/
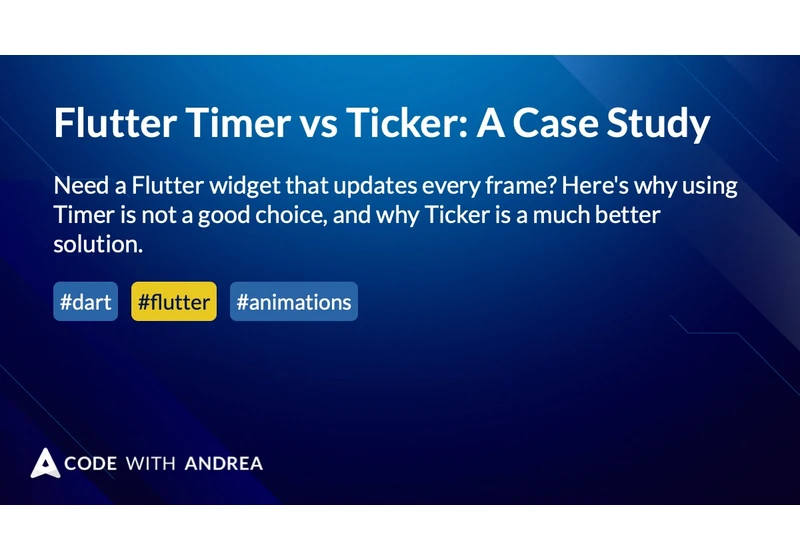
Need a Flutter widget that updates every frame? Here's why using Timer is not a good choice, and why Ticker is a much better solution. https://codewithandrea.com/articles/flutter-timer-vs-ticker/

Tired of writing JSON parsing code by hand? Here's how to automate this with code generation and the Freezed package. https://codewithandrea.com/articles/parse-json-dart-codegen-freezed/

Learn how to parse JSON and define strongly-typed model classes that can handle validation, nullable/optional values, and complex/nested JSON data. https://codewithandrea.com/articles/parse-json-dart/

Mutating state or calling async code inside the build method can cause unwanted widget rebuilds and unintended behaviour. Here are some examples and rules to follow. https://codewithandrea.com/articles/side-effects-flutter/
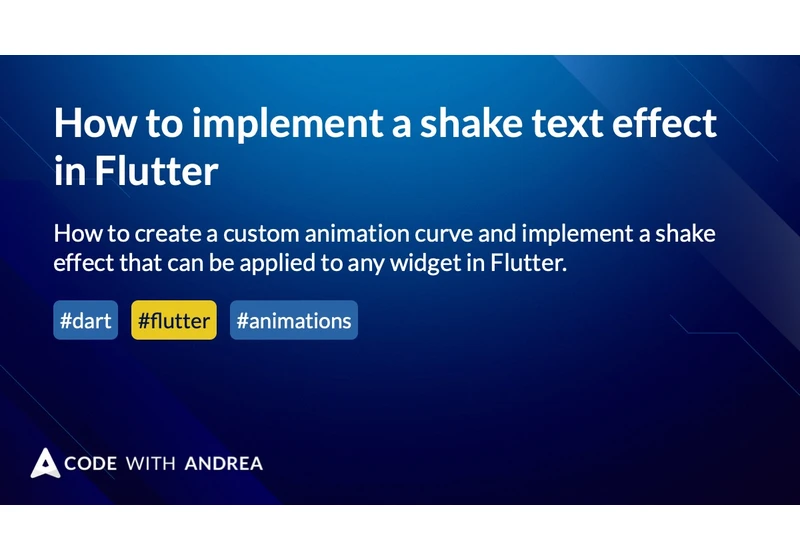
How to create a custom animation curve and implement a shake effect that can be applied to any widget in Flutter. https://codewithandrea.com/articles/shake-text-effect-flutter/
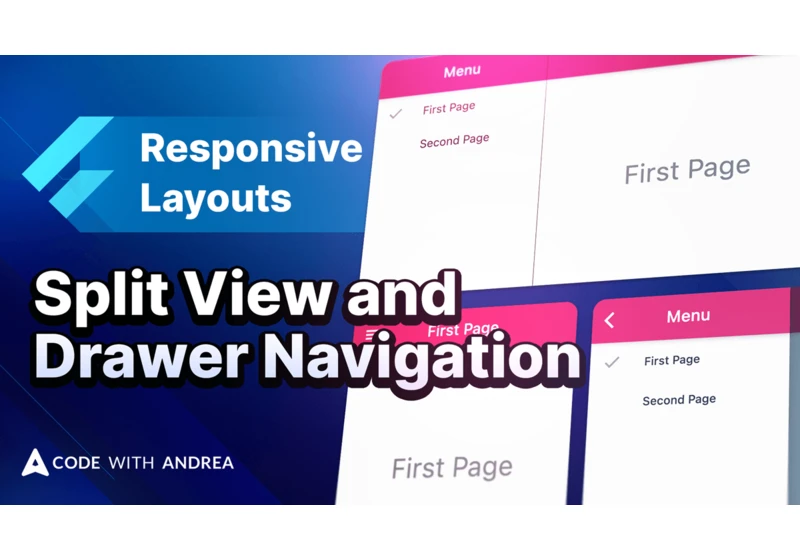
How to implement a responsive layout in Flutter by using a split view on large screens and drawer navigation on mobile. https://codewithandrea.com/articles/flutter-responsive-layouts-split-view-drawer-navigation/
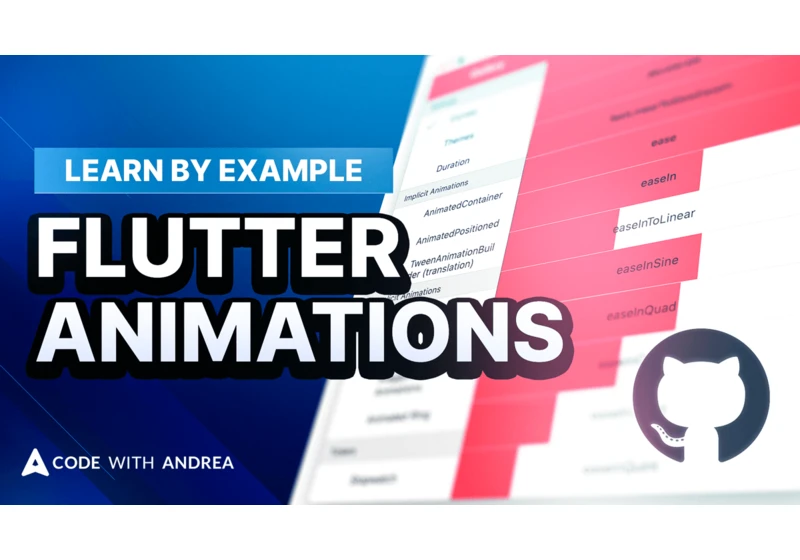
Learn how to use the most common Flutter animation APIs with examples and a free gallery app on GitHub. https://codewithandrea.com/videos/learn-flutter-animations/